Patriot CTF 2023 (Part 2)
·3 mins
Table of Contents
Introduction #
This is a continuation of the Patriot CTF write-up. If you have not checked the previous blog, be sure to check it here
1. Python XOR #
Challenge description #
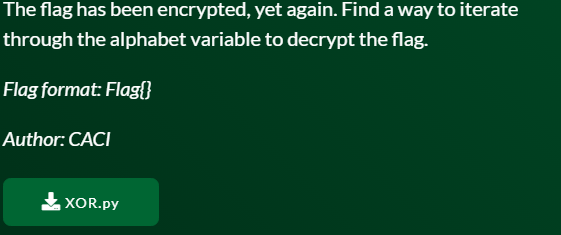
- We are given a file
XOR.py
with the following Python code:
from string import punctuation
alphabet = list(punctuation)
data = "bHEC_T]PLKJ{MW{AdW]Y"
def main():
# For loop goes here
decrypted = ''.join([chr(ord(x) ^ ord(key)) for x in data])
print(f"Key: {key}, Decrypted: {decrypted}")
main()
Source Code Analysis #
This code attempts to decrypt a string data
using an XOR cipher, but it’s missing a key and a loop for trying different keys. Here’s a brief breakdown:
- Imports punctuation characters from the
string
module.
from string import punctuation
- Creates an
alphabet
list containing all punctuation characters.
alphabet = list(punctuation)
- Defines the
data
which represents the encrypted message.
data = "bHEC_T]PLKJ{MW{AdW]Y"
- Defines a function
main
.
def main():
- Inside the main function, we are supposed to have a for loop that iterates over each character in alphabet.
- Decryption logic using a list comprehension:
decrypted = ''.join([chr(ord(x) ^ ord(key)) for x in data])
Here, ord(x)
gets the ASCII value of the character x
, and ord(key)
gets the ASCII value of the key
. The ^
operator performs a bitwise XOR between these values. chr()
then converts the result back to a character. This process is done for each character x
in data
.
6. Printing the decryption result:
print(f"Key: {key}, Decrypted: {decrypted}")
The result is printed showing the key used for decryption and the decrypted string.
- Calling the main function:
main()
= However, the code is incomplete because the for loop to iterate over each character in
- To make the code functional, we should add the for loop
from string import punctuation
alphabet = list(punctuation)
data = "bHEC_T]PLKJ{MW{AdW]Y"
def main():
for key in alphabet:
decrypted = ''.join([chr(ord(x) ^ ord(key)) for x in data])
print(f"Key: {key}, Decrypted: {decrypted}")
main()
- Adding the for loop, and running the code, we get our flag using “$” key
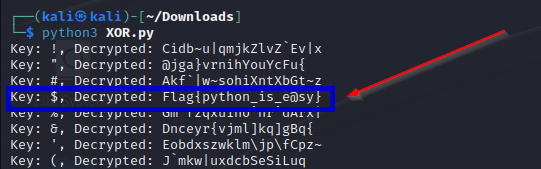
- That was easy :)
2. Uh Oh #
Challenge description #
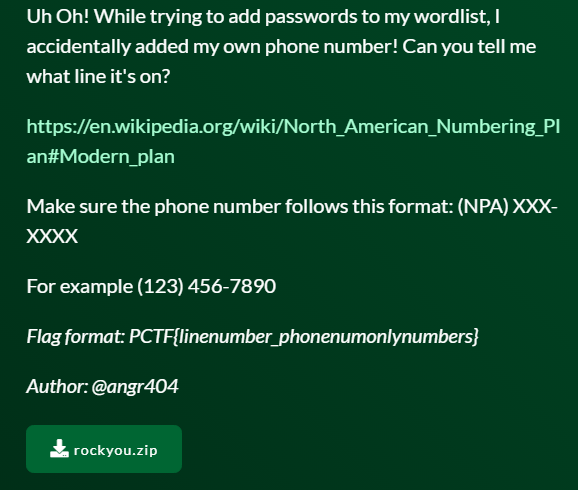
Solution #
- We can search for the phone number in the file using a regex like this
\(\d{3}\) \d{3}-\d{4}
. - To determine the line number, we can count with Python.
- Our Python script
#!/usr/bin/python
import re
# Specify the path to your text file containing passwords
file_path = "rockyou.txt"
# Define the regular expression pattern
phone_pattern = r'\(\d{3}\) \d{3}-\d{4}'
# Open the file and search for matching phone numbers
with open(file_path, 'r') as file:
lines = file.readlines()
# Initialize a line counter
line_number = 0
# Search for matching phone numbers and print line numbers
for line in lines:
line_number += 1
matches = re.findall(phone_pattern, line)
for match in matches:
print("Line {}: {}".format(line_number, match))
- And we get the line number and the phone number
Conclusion #
If you enjoyed the content, feel free to share this with others :)